Static Classes and Methods in Python
Python's object-oriented paradigm offers a diverse range of class types, including static classes and methods. Understanding and leveraging these features can significantly enhance the organization and functionality of your Python codebase. In this comprehensive guide, we'll delve deep into the concepts of static classes and methods in Python, covering their syntax, usage, and the myriad benefits they bring to your programming projects.
Understanding Python Static Classes
Static classes in Python serve as containers for functionality without the need for instantiation. Defined using the @staticmethod
decorator, these classes allow methods to be accessed directly from the class itself. Unlike conventional classes, static classes don't rely on instance-specific data, making them versatile tools for modular code organization.
Creating and Using Static Methods
Defining a static method within a class is as simple as decorating the method with @staticmethod
. These methods can then be invoked directly from the class, eliminating the need for instance creation. This versatility makes static methods ideal for grouping utility functions, factory methods, and other class-level operations.
class MathUtils:
@staticmethod
def add(x, y):
return x + y
@staticmethod
def subtract(x, y):
return x - y
# Example usage
print(MathUtils.add(3, 5)) # Output: 8
print(MathUtils.subtract(10, 4)) # Output: 6
Static methods offer a succinct means of encapsulating functionality, promoting code reuse and maintainability across projects.
Benefits of Static Methods
Static methods in Python offer a plethora of advantages, including:
- Utility Functions: Group related functions within a class without instantiation.
- Factory Methods: Simplify object creation logic within the class.
- Caching and Memoization: Store and reuse calculated results for improved performance.
- Code Organization: Organize functions under a single class for better readability and maintainability.
class StringUtils:
@staticmethod
def reverse_string(string):
return string[::-1]
By identifying these scenarios, you can effectively harness the power of static methods to enhance code structure and maintainability in your Python projects.
Static Variables in Classes
In addition to static methods, Python also supports static variables within classes. These class-level attributes are shared among all instances and retain their value across different objects. Static variables provide a convenient means of managing shared data or configuration within a class.
class Car:
count = 0
def __init__(self, name):
self.name = name
Car.count += 1
# Accessing static variable
print("Total cars:", Car.count) # Output: Total cars: 0
Static variables are invaluable for maintaining shared state across class instances, offering a streamlined approach to managing class-level data.
Conclusion
In conclusion, Python's static classes and methods offer powerful tools for organizing and encapsulating functionality within your codebase. By leveraging static methods and variables, you can promote code reusability, improve maintainability, and create more structured and readable Python programs. Whether you're grouping utility functions, creating factory methods, or managing shared class state, static classes and methods provide a versatile solution for various programming challenges.
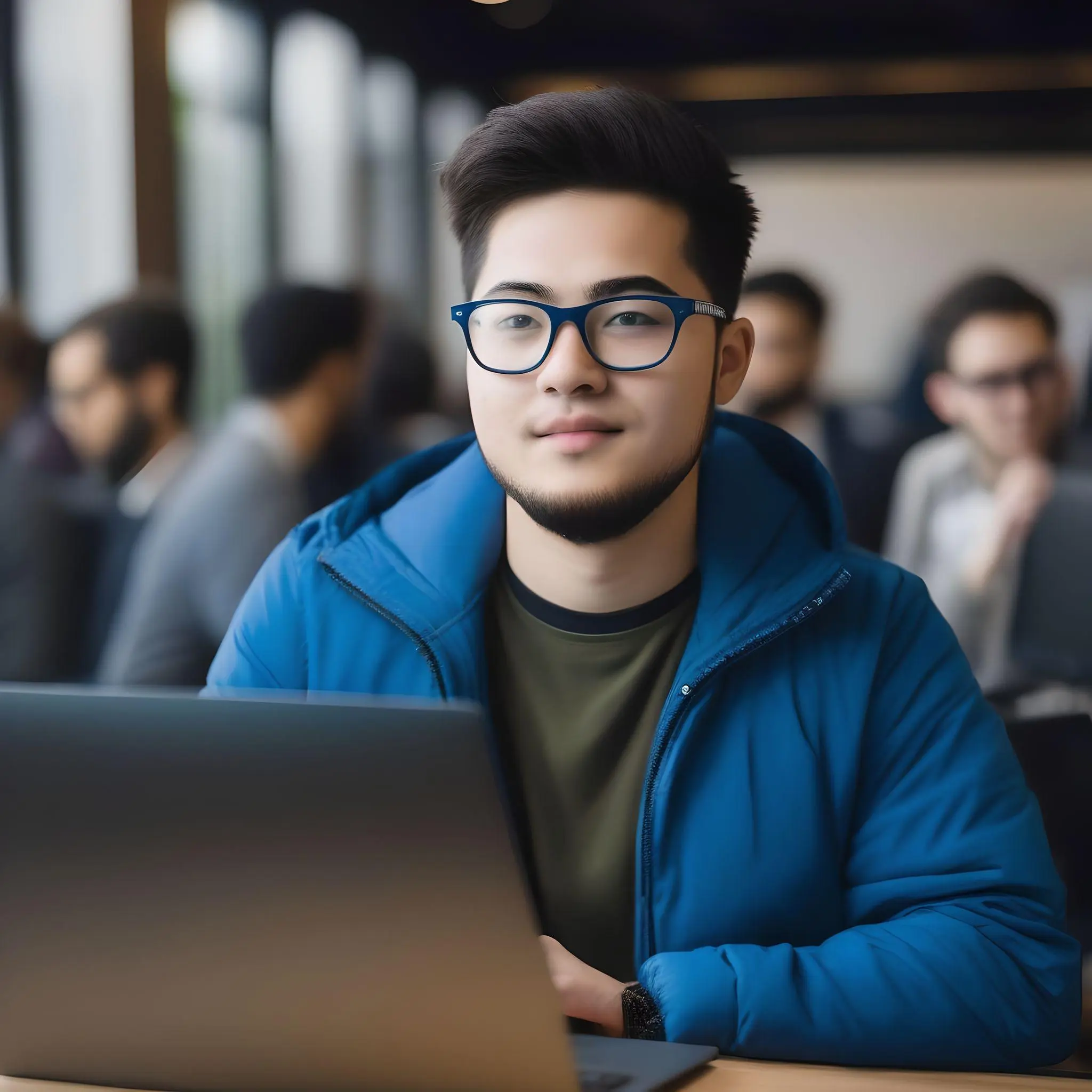
Andrew Thompson
I'm a Python developer based in Seattle and the author of this website.
Updated: 15 March 2024
About author